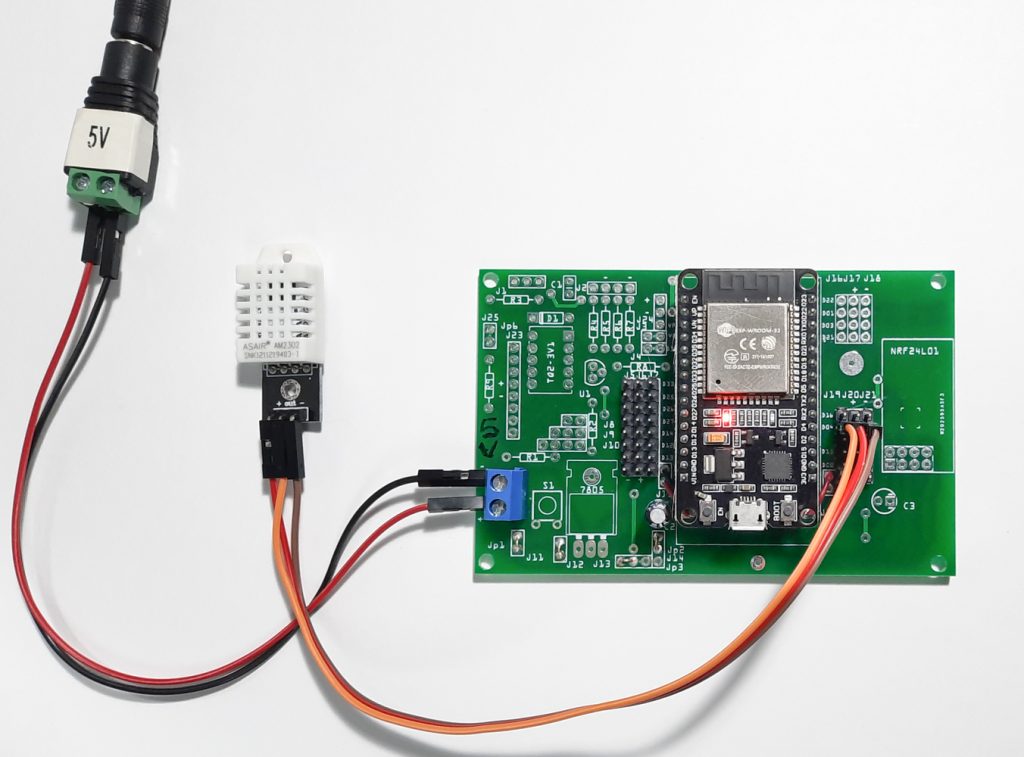
Pour le montage de la plaquette, se référer ici
Liste des composantes
- ESP32 Development Board Wireless WiFi+Bluetooth Dual Core Module WiFi Development Board for IOT
- DHT22 Single-Bus Digital Temperature and Humidity Sensor Module AM2302 Electronic Building Blocks
- 10 Pair 40 Pin 2.54mm Male Female SIL Socket Row Strip PCB Connector
- Gikfun 5.08-301-2P 2 Pin Screw Terminal Block Connector 5mm Pitch for Arduino (Pack of 20pcs) EK1601C
- Condensateur électrolytique en aluminium, 20 pièces, 35V, 10UF
Première application
Une application très simple est proposée afin de vérifier le bon fonctionnement du capteur.
La borne «out» du DHT22 est reliée sur le GPIO «D4» ( J19-D4 ) du ESP32. La borne «+» du DHT22 au «+» J20 (3,3V) et la borne «-» au «-» J21.
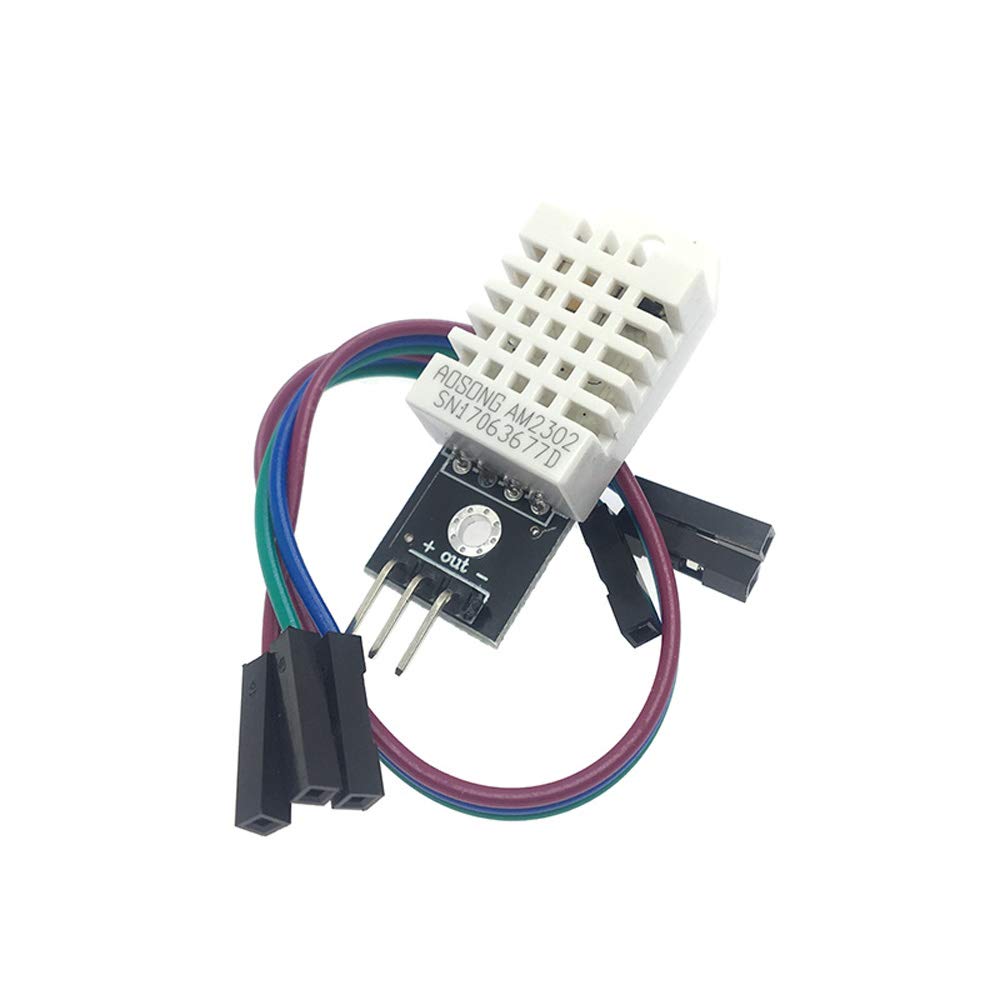
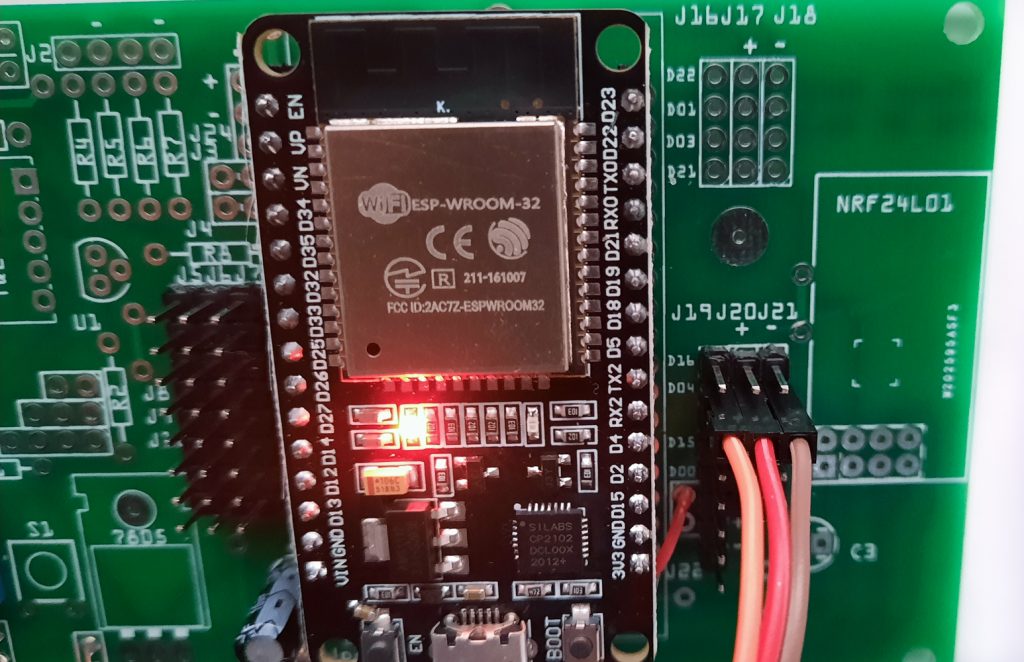
Vous trouverez le programme source et l’ensemble des explications sur le site de « RANDOM NERD TUTORIAL» à partir de ce lien :
DHT11 and DHT22 Temperature and Humidity Sensors
Remarques : La résistance n’est pas requise, le DHT22 que je propose possède déjà sa résistance.
// Example testing sketch for various DHT humidity/temperature sensors written by ladyada // REQUIRES the following Arduino libraries: // - DHT Sensor Library: https://github.com/adafruit/DHT-sensor-library // - Adafruit Unified Sensor Lib: https://github.com/adafruit/Adafruit_Sensor #include "DHT.h" #define DHTPIN 4 // Digital pin connected to the DHT sensor // Feather HUZZAH ESP8266 note: use pins 3, 4, 5, 12, 13 or 14 -- // Pin 15 can work but DHT must be disconnected during program upload. // Uncomment whatever type you're using! //#define DHTTYPE DHT11 // DHT 11 #define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321 //#define DHTTYPE DHT21 // DHT 21 (AM2301) // Connect pin 1 (on the left) of the sensor to +5V // NOTE: If using a board with 3.3V logic like an Arduino Due connect pin 1 // to 3.3V instead of 5V! // Connect pin 2 of the sensor to whatever your DHTPIN is // Connect pin 4 (on the right) of the sensor to GROUND // Connect a 10K resistor from pin 2 (data) to pin 1 (power) of the sensor // Initialize DHT sensor. // Note that older versions of this library took an optional third parameter to // tweak the timings for faster processors. This parameter is no longer needed // as the current DHT reading algorithm adjusts itself to work on faster procs. DHT dht(DHTPIN, DHTTYPE); void setup() { Serial.begin(9600); Serial.println(F("DHTxx test!")); dht.begin(); } void loop() { // Wait a few seconds between measurements. delay(2000); // Reading temperature or humidity takes about 250 milliseconds! // Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor) float h = dht.readHumidity(); // Read temperature as Celsius (the default) float t = dht.readTemperature(); // Read temperature as Fahrenheit (isFahrenheit = true) float f = dht.readTemperature(true); // Check if any reads failed and exit early (to try again). if (isnan(h) || isnan(t) || isnan(f)) { Serial.println(F("Failed to read from DHT sensor!")); return; } // Compute heat index in Fahrenheit (the default) float hif = dht.computeHeatIndex(f, h); // Compute heat index in Celsius (isFahreheit = false) float hic = dht.computeHeatIndex(t, h, false); Serial.print(F("Humidity: ")); Serial.print(h); Serial.print(F("% Temperature: ")); Serial.print(t); Serial.print(F("°C ")); Serial.print(f); Serial.print(F("°F Heat index: ")); Serial.print(hic); Serial.print(F("°C ")); Serial.print(hif); Serial.println(F("°F")); }
Deuxième application
Une deuxième application plus évoluée est proposée. Toujours avec le même montage.
Vous trouverez le programme source et l’ensemble des explications sur le site de « RANDOM NERD TUTORIAL» à partir de ce lien :
ESP32 DHT11/DHT22 Web Server – Temperature and Humidity using Arduino IDE
Remarques : La résistance n’est pas requise, le DHT22 que je propose possède déjà sa résistance.
/********* Rui Santos Complete project details at https://randomnerdtutorials.com *********/ // Import required libraries #include "WiFi.h" #include "ESPAsyncWebServer.h" #include <Adafruit_Sensor.h> #include <DHT.h> // Replace with your network credentials const char* ssid = "REPLACE_WITH_YOUR_SSID"; const char* password = "REPLACE_WITH_YOUR_PASSWORD"; #define DHTPIN 4 // Digital pin connected to the DHT sensor // Uncomment the type of sensor in use: //#define DHTTYPE DHT11 // DHT 11 #define DHTTYPE DHT22 // DHT 22 (AM2302) //#define DHTTYPE DHT21 // DHT 21 (AM2301) DHT dht(DHTPIN, DHTTYPE); // Create AsyncWebServer object on port 80 AsyncWebServer server(80); String readDHTTemperature() { // Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor) // Read temperature as Celsius (the default) float t = dht.readTemperature(); // Read temperature as Fahrenheit (isFahrenheit = true) //float t = dht.readTemperature(true); // Check if any reads failed and exit early (to try again). if (isnan(t)) { Serial.println("Failed to read from DHT sensor!"); return "--"; } else { Serial.println(t); return String(t); } } String readDHTHumidity() { // Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor) float h = dht.readHumidity(); if (isnan(h)) { Serial.println("Failed to read from DHT sensor!"); return "--"; } else { Serial.println(h); return String(h); } } const char index_html[] PROGMEM = R"rawliteral( <!DOCTYPE HTML><html> <head> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.7.2/css/all.css" integrity="sha384-fnmOCqbTlWIlj8LyTjo7mOUStjsKC4pOpQbqyi7RrhN7udi9RwhKkMHpvLbHG9Sr" crossorigin="anonymous"> <style> html { font-family: Arial; display: inline-block; margin: 0px auto; text-align: center; } h2 { font-size: 3.0rem; } p { font-size: 3.0rem; } .units { font-size: 1.2rem; } .dht-labels{ font-size: 1.5rem; vertical-align:middle; padding-bottom: 15px; } </style> </head> <body> <h2>ESP32 DHT Server</h2> <p> <i class="fas fa-thermometer-half" style="color:#059e8a;"></i> <span class="dht-labels">Temperature</span> <span id="temperature">%TEMPERATURE%</span> <sup class="units">°C</sup> </p> <p> <i class="fas fa-tint" style="color:#00add6;"></i> <span class="dht-labels">Humidity</span> <span id="humidity">%HUMIDITY%</span> <sup class="units">%</sup> </p> </body> <script> setInterval(function ( ) { var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { document.getElementById("temperature").innerHTML = this.responseText; } }; xhttp.open("GET", "/temperature", true); xhttp.send(); }, 10000 ) ; setInterval(function ( ) { var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { document.getElementById("humidity").innerHTML = this.responseText; } }; xhttp.open("GET", "/humidity", true); xhttp.send(); }, 10000 ) ; </script> </html>)rawliteral"; // Replaces placeholder with DHT values String processor(const String& var){ //Serial.println(var); if(var == "TEMPERATURE"){ return readDHTTemperature(); } else if(var == "HUMIDITY"){ return readDHTHumidity(); } return String(); } void setup(){ // Serial port for debugging purposes Serial.begin(115200); dht.begin(); // Connect to Wi-Fi WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(1000); Serial.println("Connecting to WiFi.."); } // Print ESP32 Local IP Address Serial.println(WiFi.localIP()); // Route for root / web page server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){ request->send_P(200, "text/html", index_html, processor); }); server.on("/temperature", HTTP_GET, [](AsyncWebServerRequest *request){ request->send_P(200, "text/plain", readDHTTemperature().c_str()); }); server.on("/humidity", HTTP_GET, [](AsyncWebServerRequest *request){ request->send_P(200, "text/plain", readDHTHumidity().c_str()); }); // Start server server.begin(); } void loop(){ }